Are you looking to build an advanced web application that combines the power of web technology like React and the popular CMS like WordPress? If your answer is yes, then this informational piece can help you achieve this goal. This article will discuss how to create a modern web app using React with WordPress. More precisely, we will also walk you through some more crucial processes, like:
- What are WordPress REST API and the ways to access it from your front-end
- Building a React SPA (Single Page Application) WordPress theme using this API
- Managing React Hooks and creating a custom one
But before we start building our modern web app with React and WordPress, let’s take a brief about WordPress’s Rest API and how it will make your task easy.
What is the WordPress REST API?
WordPress, being one of the popular Content Management Systems (CMS), needs no special introduction. But you might not be aware of WordPress REST API or Headless CMS, right? No worries, let’s understand this one by one.
Earlier, using CMS like WordPress meant you had to design your front-end using PHP. However, now with a headless CMS, you can create your front-end with whatever web technologies you prefer. It happens because of the separation of the front-end and the back-end with the help of an API. Therefore, a headless CMS is one that you can use as a generic content management system irrespective of the technology used to create the front-end.
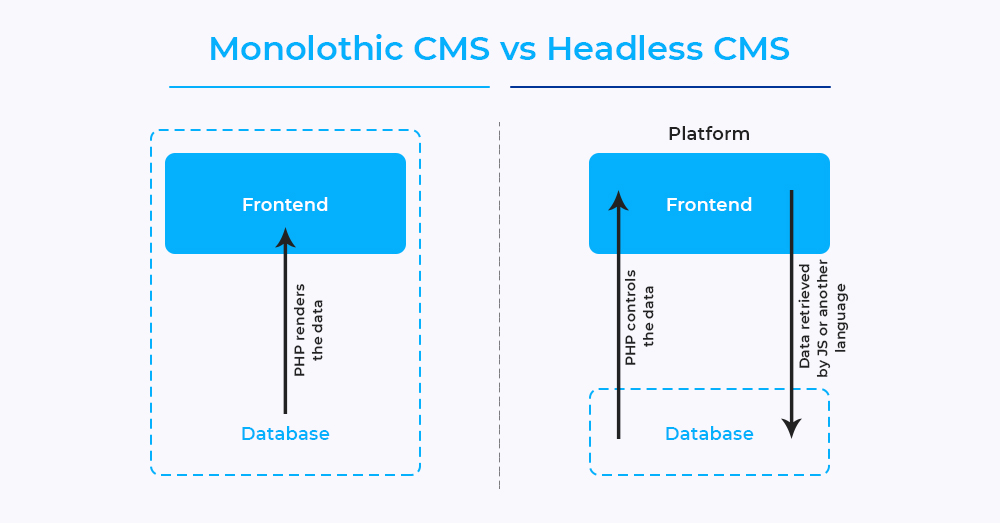
It introduced a whole new world of possibilities for everyone who does not want their front-end to be WordPress-PHP generated (Monolithic CMS). Now, you can use a wide range of front-end frameworks like React and hook them to a WordPress back-end to build websites and applications. In this way, you can quickly design beautiful SPAs using React with WordPress.
How is WordPress REST API beneficial for you?
The WordPress REST API proves to be a game-changer for WordPress.If you are looking for a content-driven solution, WordPress can be an excellent choice for back-end technology. It allows developers to design interactive plugins and themes and lets them power third-party apps with WordPress CMS.
Moreover, WordPress REST API makes it pretty easy to connect to any application. Since REST API speaks in the same language as that of cross-technology interfaces (that is, JSON), it allows developers to interact with them (interfaces) easily. It transfers the data in JSON, which is compatible with a plethora of web technologies. That’s how it helps the developers to build interactive web applications using React with WordPress.
Key Acronyms related to WordPress REST API you should know about
- CMS (Content Management System): A CMS is computer software that manages the creation and modification of digital content. WordPress, Joomla, Drupal are some examples of CMS.
- API (Application Program Interface): It is a software intermediary that lets two applications interact and is used to build software applications. It defines how you share the information between programs and helps you understand how different app components will interact with each other.
- REST (Representational State Transfer): It is an architectural style-defining constraint that provides standards between systems worldwide. When web services meet this architecture, we call them RESTful APIs or, simply, REST APIs. When someone calls a REST API, the server passes the representational state of the requested resource to the client.
- JSON (JavaScript Object Notation): It is a format derived from JavaScript (JS), used for structuring, storing, and transferring data so that many applications can read it. Its primary objective is to make interactions between something, such as a CMS (WordPress), and any application convenient.
- SPA (Single-Page Applications): A Single-Page Application loads the content dynamically in front of the user rather than loading all the new pages from the server.
Why are we using React for our project?
Well, at first, we chose it just as a choice, from all the other web technologies, present there. But since it got so popular recently, it has become one of the most preferred choices available for us. Furthermore, there is no doubt that it is one of the best frameworks to create robust and highly interactive web applications due to many of its exciting features, such as:
- Flexible and reusable component system
- Efficient workflow with JSX
- Virtual DOM
- Declarative UI
- Fast data rendering and much more
Also, one more added feature is React Hooks, which we will also use in this tutorial.
So, enough talking, let’s get started with some action now and create modern web apps using React with WordPress!
Making a modern app using React with WordPress
In this example, we are creating a SPA for a WordPress theme. Although we will not go deep into the theme’s interface and design, we will focus more on the application building part instead.
So, let’s do this!
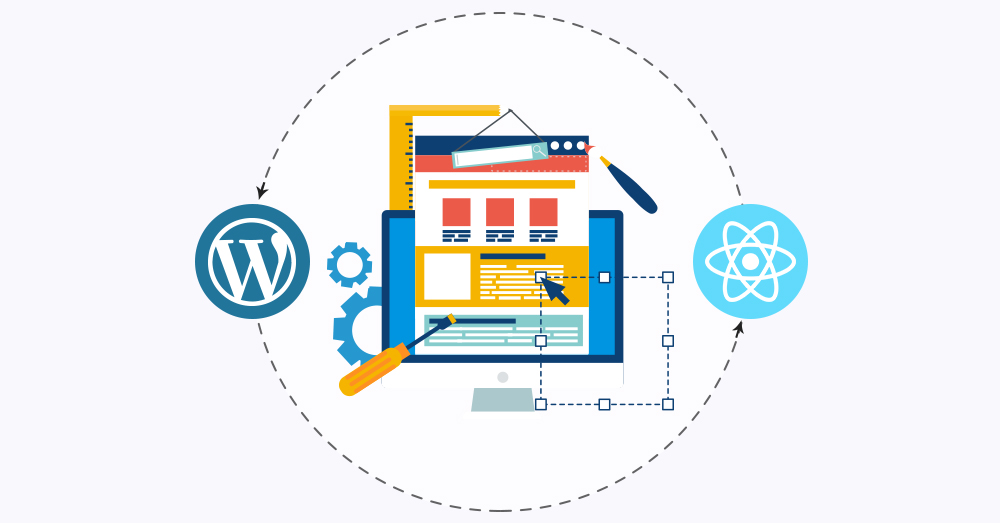
Step 1: Creating a database for WordPress and fetching the WordPress data
The first thing you need here is to have a running WordPress website. Assuming that you have already got a WordPress installation working with your local system, let’s move ahead.
In local development, we have made some changes to the wp-config.php file to make the demo run. We need to give the database name, login credentials for the database, and hostname. It will look like this:
After building the website, we have to deploy it into a hosting site such as Heroku, FireBase, AWS, Github pages, etc.
You can already use the REST API from your front-end by making a GET request to get all the posts for the user who logged into their wordpress website using ‘/wp-JSON/wp/v2/{post type}’. For example, you can get all posts from /wp-JSON/wp/v2/posts.
From our front end, the requests could be made in two ways. To call/fetch the REST API, you need a user request using the fetch or React library called Axios.
For example, using fetch, we can call the REST API like this:
fetch(‘/wp-json/wp/v2/posts’)
.then(response => response.json())
.then(posts => console.log(posts));
Using Axios, you can fetch the REST API data in this way:
axios.get(‘/wp-json/wp/v2/posts’).get(response =>response.json()).then(posts => console.log(posts));
Step 2: Creating a React project and querying the WP REST API
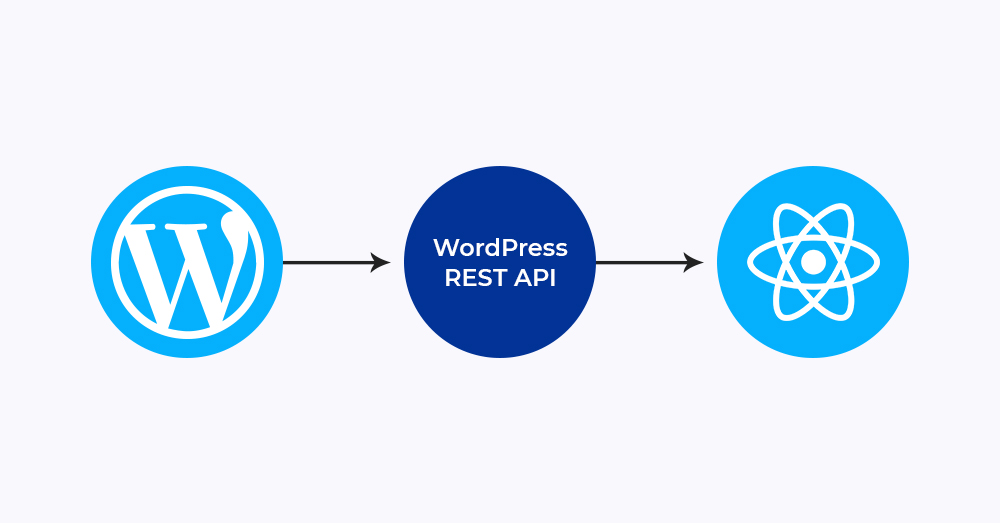
To create a React project, we need to run this command in a terminal:
npx create-react-app project-name
Note: npx is provided with NodeJS to run commands without installing them globally.
Now, add bootstrap to the project (cd project-name)
To install bootstrap, you can use this command: npm install bootstrap.
To query the posts data, add the following Posts component:
In the src folder, you need to create a file called Posts.js
Include it into App.js
import React, { useEffect, useState } from “react”;
import axios from “axios”;
function Posts() {
const [posts, setPosts] = useState([]);
useEffect(() => {
axios
.get(“http://local.wpproject.com/public_html/wp-json/wp/v2/posts”)
.then((response) => {
console.log(response)
});
}, []);
return (
<div>
</div>
)
}
export default Posts;
useState is used for a state management system with an initial value, and after the Rest API calls, we can update the state using the setPosts (response.data).
Then useEffect enables the system to run the fetch code when the component is mounted.
Finally, to render a list of posts, you can “map” over the posts array and return components for each one of them. However, there are a few things that tells you how useEffect works:
<div className=”post-container”>
{posts.length > 0 ? (
<div className=””>
{posts.map((post) => {
return <PostCard post={post} />
})}
</div>
) : (
<p>No posts found</p>
)}
</div>
Step 3: Creating a custom React Hook
To simplify this, you can create your own React Hook “useCustomHook” (in ./useCustomHook.js)
import { useEffect, useState } from ‘react’;
export default function useCustomHook(url) {
const [data, setData] = useState(null);
useEffect(() => {
async function loadData() {
const response = await fetch(url);
if(!response.ok) {
// oups! something went wrong
return;
}
const posts = await response.json();
setData(posts);
}
loadData();
}, [url]);
return data;
}
Final Thoughts
WordPress is constantly evolving, and so is React. They both are great, so why not combine the two? The JS-based approach is already spending things in the WordPress space. Their combined power can help you build a robust modern web app with advanced technologies. This article has tried to provide you with an overview to develop an interactive web app using React with WordPress. If the concept is clear and you like it, go check it out and create a high-performing web app quickly and hassle-free.